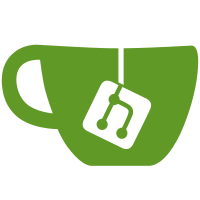
* Second websocket channel for the projector * Removed use of projector requirements for REST API requests. Refactored data serializing for projector websocket connection. * Refactor the way that the projector autoupdate get its data. * Fixed missing assignment slide title for hidden items. * Release all items for item list slide and list of speakers slide. Fixed error with motion workflow. * Created CollectionElement class which helps to handle autoupdate.
47 lines
1.4 KiB
Python
47 lines
1.4 KiB
Python
from ..utils.access_permissions import BaseAccessPermissions
|
|
|
|
|
|
class ItemAccessPermissions(BaseAccessPermissions):
|
|
"""
|
|
Access permissions container for Item and ItemViewSet.
|
|
"""
|
|
def check_permissions(self, user):
|
|
"""
|
|
Returns True if the user has read access model instances.
|
|
"""
|
|
return user.has_perm('agenda.can_see')
|
|
|
|
def get_serializer_class(self, user=None):
|
|
"""
|
|
Returns serializer class.
|
|
"""
|
|
from .serializers import ItemSerializer
|
|
|
|
return ItemSerializer
|
|
|
|
# TODO: In the following method we use full_data['is_hidden'] but this can be out of date.
|
|
|
|
def get_restricted_data(self, full_data, user):
|
|
"""
|
|
Returns the restricted serialized data for the instance prepared
|
|
for the user.
|
|
"""
|
|
if (user.has_perm('agenda.can_see') and
|
|
(not full_data['is_hidden'] or
|
|
user.has_perm('agenda.can_see_hidden_items'))):
|
|
data = full_data
|
|
else:
|
|
data = None
|
|
return data
|
|
|
|
def get_projector_data(self, full_data):
|
|
"""
|
|
Returns the restricted serialized data for the instance prepared
|
|
for the projector. Removes field 'comment'.
|
|
"""
|
|
data = {}
|
|
for key in full_data.keys():
|
|
if key != 'comment':
|
|
data[key] = full_data[key]
|
|
return data
|