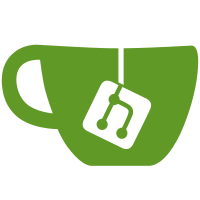
Sorts the Workflow table to be more predictable Adds new shared SCSS table rules. Adds a default with as 100% (there have never been half tables) Overwrites the rules for sticky tables
50 lines
1.3 KiB
TypeScript
50 lines
1.3 KiB
TypeScript
import { BaseModel } from '../base/base-model';
|
|
import { WorkflowState } from './workflow-state';
|
|
|
|
/**
|
|
* Representation of a motion workflow. Has the nested property 'states'
|
|
* @ignore
|
|
*/
|
|
export class Workflow extends BaseModel<Workflow> {
|
|
public static COLLECTIONSTRING = 'motions/workflow';
|
|
|
|
public id: number;
|
|
public name: string;
|
|
public states: WorkflowState[];
|
|
public first_state_id: number;
|
|
|
|
public constructor(input?: any) {
|
|
super(Workflow.COLLECTIONSTRING, input);
|
|
}
|
|
|
|
/**
|
|
* Check if the containing @link{WorkflowState}s contain a given ID
|
|
* @param id The State ID
|
|
*/
|
|
public isStateContained(obj: number | WorkflowState): boolean {
|
|
let id: number;
|
|
if (obj instanceof WorkflowState) {
|
|
id = obj.id;
|
|
} else {
|
|
id = obj;
|
|
}
|
|
|
|
return this.states.some(state => state.id === id);
|
|
}
|
|
|
|
/**
|
|
* Sorts the states of this workflow
|
|
*/
|
|
public sortStates(): void {
|
|
this.states.sort((a, b) => (a.id > b.id ? 1 : -1));
|
|
}
|
|
|
|
public deserialize(input: any): void {
|
|
Object.assign(this, input);
|
|
|
|
if (input.states instanceof Array) {
|
|
this.states = input.states.map(workflowStateData => new WorkflowState(workflowStateData));
|
|
}
|
|
}
|
|
}
|