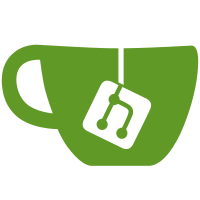
Also: Set linting output to "stylish" (more readable error messages in terminal output) Remove 2nd linting output rename "appOsPerms" to just "osPerms" including filename and classname rename all selectors from "app" to "os"
34 lines
921 B
TypeScript
34 lines
921 B
TypeScript
import { Directive, Output, EventEmitter, ElementRef, OnDestroy } from '@angular/core';
|
|
|
|
/**
|
|
* detects changes in DOM and emits a signal on changes.
|
|
*
|
|
* @example (appDomChange)="onChange($event)"
|
|
*/
|
|
@Directive({
|
|
selector: '[osDomChange]'
|
|
})
|
|
export class DomChangeDirective implements OnDestroy {
|
|
private changes: MutationObserver;
|
|
|
|
@Output() public domChange = new EventEmitter();
|
|
|
|
public constructor(private elementRef: ElementRef) {
|
|
const element = this.elementRef.nativeElement;
|
|
|
|
this.changes = new MutationObserver((mutations: MutationRecord[]) => {
|
|
mutations.forEach((mutation: MutationRecord) => this.domChange.emit(mutation));
|
|
});
|
|
|
|
this.changes.observe(element, {
|
|
attributes: true,
|
|
childList: true,
|
|
characterData: true
|
|
});
|
|
}
|
|
|
|
public ngOnDestroy(): void {
|
|
this.changes.disconnect();
|
|
}
|
|
}
|