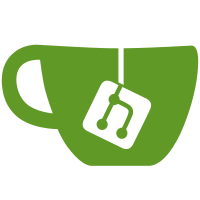
- cleaned up a lot of code - removed required majotiry from forms - renamed verbose "Majority method" to "Required majority" - poll-progress-bar only counts present user - enhanced motion poll tile chart layout - removed PercentBase.Votes - added pollPercentBase pipe - Show the voting percent next to chart in motion detail - change the head bar to "Voting is open" and "Ballot is open" - merged the voting configs to their corresponding config-categories - re-add ballot paper configs - Add "more" button to motion polls - Adjusted the motion results table - Hide entries without information - Show icons for Y N A - Show percentage next to Y N A
37 lines
1.0 KiB
TypeScript
37 lines
1.0 KiB
TypeScript
import { Pipe, PipeTransform } from '@angular/core';
|
|
|
|
import { ViewBasePoll } from 'app/site/polls/models/view-base-poll';
|
|
|
|
/**
|
|
* Uses a number and a ViewPoll-object.
|
|
* Converts the number to the voting percent base using the
|
|
* given 100%-Base option in the poll object
|
|
*
|
|
* returns null if a percent calculation is not possible
|
|
* or the result is 0
|
|
*
|
|
* @example
|
|
* ```html
|
|
* <span> {{ voteYes | pollPercentBase: poll }} </span>
|
|
* ```
|
|
*/
|
|
@Pipe({
|
|
name: 'pollPercentBase'
|
|
})
|
|
export class PollPercentBasePipe implements PipeTransform {
|
|
private decimalPlaces = 3;
|
|
|
|
public transform(value: number, viewPoll: ViewBasePoll): string | null {
|
|
const totalByBase = viewPoll.getPercentBase();
|
|
|
|
if (totalByBase) {
|
|
const percentNumber = (value / totalByBase) * 100;
|
|
if (percentNumber > 0) {
|
|
const result = percentNumber % 1 === 0 ? percentNumber : percentNumber.toFixed(this.decimalPlaces);
|
|
return `(${result}%)`;
|
|
}
|
|
}
|
|
return null;
|
|
}
|
|
}
|