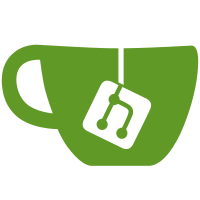
pg_isready was not connecting to the configured database or as the configured user. While that did not cause problems here, it did trigger error messages on the database side.
87 lines
2.4 KiB
Bash
Executable File
87 lines
2.4 KiB
Bash
Executable File
#!/bin/bash
|
|
|
|
set -e
|
|
|
|
warn_insecure_admin() {
|
|
cat <<-EOF
|
|
|
|
==============================================
|
|
WARNING
|
|
==============================================
|
|
|
|
WARNING: INSECURE ADMIN ACCOUNT CONFIGURATION!
|
|
|
|
EOF
|
|
sleep 10
|
|
}
|
|
|
|
# Set DJANGO_SECRET_KEY variable
|
|
source /run/secrets/django || true
|
|
[[ -n "$DJANGO_SECRET_KEY" ]] || {
|
|
echo "ERROR: Django secret key undefined! Cannot continue."
|
|
sleep 5
|
|
exit 2
|
|
}
|
|
export SECRET_KEY="$DJANGO_SECRET_KEY"
|
|
|
|
export PGHOST="${DATABASE_HOST:-pgbouncer}"
|
|
export PGPORT="${DATABASE_PORT:-5432}"
|
|
export PGDATABASE="${DATABASE_NAME:-openslides}"
|
|
export PGUSER="${DATABASE_USER:-openslides}"
|
|
until pg_isready; do
|
|
echo "Waiting for Postgres cluster to become available..."
|
|
sleep 3
|
|
done
|
|
|
|
# Wait for redis
|
|
wait-for-it "${REDIS_HOST:-redis}:${REDIS_PORT:-6379}"
|
|
wait-for-it "${REDIS_SLAVE_HOST:-redis-slave}:${REDIS_SLAVE_PORT:-6379}"
|
|
|
|
echo 'running migrations'
|
|
python -u manage.py migrate
|
|
|
|
# Admin
|
|
if [[ -f /run/secrets/os_admin ]]; then
|
|
echo "Retrieving secure admin password"
|
|
source /run/secrets/os_admin
|
|
if [[ -n "${OPENSLIDES_ADMIN_PASSWORD}" ]]; then
|
|
echo "Changing admin password"
|
|
python manage.py changedefaultadminpassword "${OPENSLIDES_ADMIN_PASSWORD}"
|
|
else
|
|
warn_insecure_admin
|
|
fi
|
|
else
|
|
warn_insecure_admin
|
|
fi
|
|
|
|
# Main user
|
|
if [[ -f /run/secrets/os_user ]]; then
|
|
echo "Retrieving secure user credentials"
|
|
source /run/secrets/os_user
|
|
if [[ -n "${OPENSLIDES_USER_FIRSTNAME}" ]] &&
|
|
[[ -n "${OPENSLIDES_USER_LASTNAME}" ]] &&
|
|
[[ -n "${OPENSLIDES_USER_PASSWORD}" ]]; then
|
|
user_name="${OPENSLIDES_USER_FIRSTNAME} ${OPENSLIDES_USER_LASTNAME}"
|
|
echo "Adding user: ${user_name}"
|
|
# createopenslidesuser: error: the following arguments are required:
|
|
# first_name, last_name, username, password, groups_id
|
|
# email is optional
|
|
# userid forces to to only create a user with this id, if it not exists before.
|
|
python manage.py createinitialuser \
|
|
--email "${OPENSLIDES_USER_EMAIL:-}" \
|
|
"${OPENSLIDES_USER_FIRSTNAME}" \
|
|
"${OPENSLIDES_USER_LASTNAME}" \
|
|
"${user_name}" \
|
|
"${OPENSLIDES_USER_PASSWORD}" \
|
|
2
|
|
else
|
|
echo "Incomplete user account data. Skipping account creation."
|
|
fi
|
|
fi
|
|
|
|
# SAML setup
|
|
. /usr/local/lib/saml-setup.sh
|
|
|
|
echo "Done migrating and setting up user accounts..."
|
|
python -m http.server --directory /app/empty --bind 0.0.0.0 8000
|