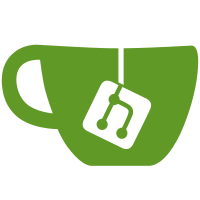
* Activate restricted_data_cache on inmemory cache * Use ElementCache in rest-api get requests * Get requests on the restapi return 404 when the user has no permission * Added async function for has_perm and in_some_groups * changed Cachable.get_restricted_data to be an ansync function * rewrote required_user_system * changed default implementation of access_permission.check_permission to check a given permission or check if anonymous is enabled
44 lines
1.7 KiB
Python
44 lines
1.7 KiB
Python
from django.apps import apps
|
|
from django.contrib.auth.models import Permission
|
|
from django.contrib.contenttypes.models import ContentType
|
|
from django.db.models import Q
|
|
from django.dispatch import Signal
|
|
|
|
|
|
# This signal is send when the migrate command is done. That means it is sent
|
|
# after post_migrate sending and creating all Permission objects. Don't use it
|
|
# for other things than dealing with Permission objects.
|
|
post_permission_creation = Signal()
|
|
|
|
# This signal is sent if a permission is changed (e. g. a group gets a new
|
|
# permission). Connected receivers may yield Collections.
|
|
permission_change = Signal()
|
|
|
|
|
|
def delete_django_app_permissions(sender, **kwargs):
|
|
"""
|
|
Deletes the permissions, Django creates by default. Only required
|
|
for auth, contenttypes and sessions.
|
|
"""
|
|
contenttypes = ContentType.objects.filter(
|
|
Q(app_label='auth') |
|
|
Q(app_label='contenttypes') |
|
|
Q(app_label='sessions'))
|
|
Permission.objects.filter(content_type__in=contenttypes).delete()
|
|
|
|
|
|
def get_permission_change_data(sender, permissions, **kwargs):
|
|
"""
|
|
Yields all necessary Cachables if the respective permissions change.
|
|
"""
|
|
core_app = apps.get_app_config(app_label='core')
|
|
for permission in permissions:
|
|
if permission.content_type.app_label == core_app.label:
|
|
if permission.codename == 'can_see_projector':
|
|
yield core_app.get_model('Projector')
|
|
elif permission.codename == 'can_manage_projector':
|
|
yield core_app.get_model('ProjectorMessage')
|
|
yield core_app.get_model('Countdown')
|
|
elif permission.codename == 'can_use_chat':
|
|
yield core_app.get_model('ChatMessage')
|