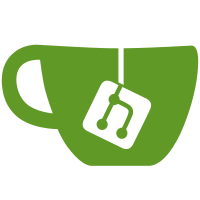
* Activate restricted_data_cache on inmemory cache * Use ElementCache in rest-api get requests * Get requests on the restapi return 404 when the user has no permission * Added async function for has_perm and in_some_groups * changed Cachable.get_restricted_data to be an ansync function * rewrote required_user_system * changed default implementation of access_permission.check_permission to check a given permission or check if anonymous is enabled
38 lines
1008 B
Python
38 lines
1008 B
Python
from typing import Any
|
|
|
|
from django.conf import settings
|
|
|
|
|
|
try:
|
|
import aioredis
|
|
except ImportError:
|
|
use_redis = False
|
|
else:
|
|
# set use_redis to true, if there is a value for REDIS_ADDRESS in the settings
|
|
redis_address = getattr(settings, 'REDIS_ADDRESS', '')
|
|
use_redis = bool(redis_address)
|
|
|
|
|
|
class RedisConnectionContextManager:
|
|
"""
|
|
Async context manager for connections
|
|
"""
|
|
# TODO: contextlib.asynccontextmanager can be used in python 3.7
|
|
|
|
def __init__(self, redis_address: str) -> None:
|
|
self.redis_address = redis_address
|
|
|
|
async def __aenter__(self) -> 'aioredis.RedisConnection':
|
|
self.conn = await aioredis.create_redis(self.redis_address)
|
|
return self.conn
|
|
|
|
async def __aexit__(self, exc_type: Any, exc: Any, tb: Any) -> None:
|
|
self.conn.close()
|
|
|
|
|
|
def get_connection() -> RedisConnectionContextManager:
|
|
"""
|
|
Returns contextmanager for a redis connection.
|
|
"""
|
|
return RedisConnectionContextManager(redis_address)
|