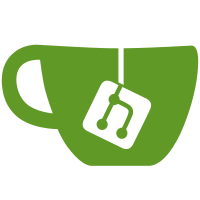
Changes after some reviews are done. Problematic is still that the JS can not be moved to an extra file because of the template tags in the code.
50 lines
1.8 KiB
Python
50 lines
1.8 KiB
Python
#!/usr/bin/env python
|
||
# -*- coding: utf-8 -*-
|
||
"""
|
||
openslides.agenda.signals
|
||
~~~~~~~~~~~~~~~~~~~~~~~~~
|
||
|
||
Signals for the agenda app.
|
||
|
||
:copyright: (c) 2011–2013 by the OpenSlides team, see AUTHORS.
|
||
:license: GNU GPL, see LICENSE for more details.
|
||
"""
|
||
|
||
from django.dispatch import receiver
|
||
from django import forms
|
||
from django.utils.translation import ugettext_lazy, ugettext_noop, ugettext as _
|
||
|
||
from openslides.config.signals import config_signal
|
||
from openslides.config.api import ConfigVariable, ConfigPage
|
||
|
||
|
||
# TODO: Reinsert the datepicker scripts in the template
|
||
|
||
@receiver(config_signal, dispatch_uid='setup_agenda_config_page')
|
||
def setup_agenda_config_page(sender, **kwargs):
|
||
"""
|
||
Agenda config variables.
|
||
"""
|
||
# TODO: Insert validator for the format or use other field carefully.
|
||
agenda_start_event_date_time = ConfigVariable(
|
||
name='agenda_start_event_date_time',
|
||
default_value='',
|
||
form_field=forms.CharField(
|
||
widget=forms.DateTimeInput(format='%d.%m.%Y %H:%M'),
|
||
required=False,
|
||
label=ugettext_lazy('Begin of event'),
|
||
help_text=_('Input format: DD.MM.YYYY HH:MM')))
|
||
|
||
extra_stylefiles = ['styles/timepicker.css', 'styles/jquery-ui/jquery-ui.custom.min.css']
|
||
extra_javascript = ['javascript/jquery-ui.custom.min.js',
|
||
'javascript/jquery-ui-timepicker-addon.min.js',
|
||
'javascript/jquery-ui-sliderAccess.min.js']
|
||
|
||
return ConfigPage(title=ugettext_noop('Agenda'),
|
||
url='agenda',
|
||
required_permission='config.can_manage',
|
||
weight=20,
|
||
variables=(agenda_start_event_date_time,),
|
||
extra_context={'extra_stylefiles': extra_stylefiles,
|
||
'extra_javascript': extra_javascript})
|