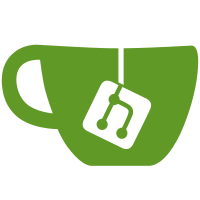
* Second websocket channel for the projector * Removed use of projector requirements for REST API requests. Refactored data serializing for projector websocket connection. * Refactor the way that the projector autoupdate get its data. * Fixed missing assignment slide title for hidden items. * Release all items for item list slide and list of speakers slide. Fixed error with motion workflow. * Created CollectionElement class which helps to handle autoupdate.
57 lines
1.6 KiB
Python
57 lines
1.6 KiB
Python
from django.db import models
|
|
|
|
|
|
class MinMaxIntegerField(models.IntegerField):
|
|
"""
|
|
IntegerField with options to set a min- and a max-value.
|
|
"""
|
|
|
|
def __init__(self, min_value=None, max_value=None, *args, **kwargs):
|
|
self.min_value, self.max_value = min_value, max_value
|
|
super(MinMaxIntegerField, self).__init__(*args, **kwargs)
|
|
|
|
def formfield(self, **kwargs):
|
|
defaults = {'min_value': self.min_value, 'max_value': self.max_value}
|
|
defaults.update(kwargs)
|
|
return super(MinMaxIntegerField, self).formfield(**defaults)
|
|
|
|
|
|
class RESTModelMixin:
|
|
"""
|
|
Mixin for Django models which are used in our REST API.
|
|
"""
|
|
|
|
access_permissions = None
|
|
|
|
def get_root_rest_element(self):
|
|
"""
|
|
Returns the root rest instance.
|
|
|
|
Uses self as default.
|
|
"""
|
|
return self
|
|
|
|
@classmethod
|
|
def get_access_permissions(cls):
|
|
"""
|
|
Returns a container to handle access permissions for this model and
|
|
its corresponding viewset.
|
|
"""
|
|
return cls.access_permissions
|
|
|
|
@classmethod
|
|
def get_collection_string(cls):
|
|
"""
|
|
Returns the string representing the name of the collection. Returns
|
|
None if this is not a so called root rest instance.
|
|
"""
|
|
# TODO Check if this is a root rest element class and return None if not.
|
|
return '/'.join((cls._meta.app_label.lower(), cls._meta.object_name.lower()))
|
|
|
|
def get_rest_pk(self):
|
|
"""
|
|
Returns the primary key used in the REST API. By default this is
|
|
the database pk.
|
|
"""
|
|
return self.pk
|