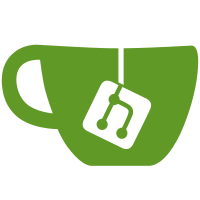
- moved all server related things into the folder `server`, so this configuration is parallel to the client. - All main "services" are now folders in the root directory - Added Dockerfiles to each service (currently server and client) - Added a docker compose configuration to start everything together. Currently there are heavy dependencies into https://github.com/OpenSlides/openslides-docker-compose - Resturctured the .gitignore. If someone needs something excluded, please add it to the right section. - Added initial build setup with Docker and docker-compose. - removed setup.py. We won't deliver OpenSlides via pip anymore.
84 lines
3.4 KiB
Python
84 lines
3.4 KiB
Python
# Generated by Django 2.1 on 2018-08-31 13:17
|
|
|
|
import django.db.models.deletion
|
|
import jsonfield.encoder
|
|
import jsonfield.fields
|
|
from django.db import migrations, models
|
|
|
|
|
|
class Migration(migrations.Migration):
|
|
"""
|
|
This is a series of logically connected migrations that needs to be in separate
|
|
files. See issue #4266 and https://docs.djangoproject.com/en/2.1/ref/migration-operations/
|
|
|
|
`On databases that do support DDL transactions (SQLite and PostgreSQL), RunPython operations
|
|
do not have any transactions automatically added besides the transactions created for
|
|
each migration. Thus, on PostgreSQL, for example, you should avoid combining schema
|
|
changes and RunPython operations in the same migration or you may hit errors like
|
|
OperationalError: cannot ALTER TABLE "mytable" because it has pending trigger events.`
|
|
|
|
Because we need some scheam changes, copy data, schema changes, copy data, ..., we need
|
|
to split this up.
|
|
|
|
Goal: Remove the motion_version. Move all fields to the motion model and keep all data.
|
|
This also affects change recommendations, that are linked to versions, not motions. All
|
|
change recommendations that are connected to active motions versions must be kept, too.
|
|
|
|
|
|
What is done (migration file):
|
|
- Create Title, Text, ... fields and new foreign key from CRs to motion (1)
|
|
- Copy data from active version to the motion model (2)
|
|
- Migrate change recommendations (2)
|
|
- Cleanup1: remove all unnecessary fields and delete motion version model (3)
|
|
- Cleanup2: alter some other fields that must be in a seperate migration file (Idk why..) (4)
|
|
"""
|
|
|
|
dependencies = [("motions", "0010_auto_20180822_1042")]
|
|
|
|
operations = [
|
|
# Create new fields. Title and Text have empty defaults, but the values
|
|
# should be overwritten by copy_motion_version_content_to_motion in migration step (2).
|
|
# In the last migration step these defaults are removed.
|
|
migrations.AddField(
|
|
model_name="motion",
|
|
name="title",
|
|
field=models.CharField(max_length=255, default=""),
|
|
),
|
|
migrations.AddField(
|
|
model_name="motion", name="text", field=models.TextField(default="")
|
|
),
|
|
migrations.AddField(
|
|
model_name="motion",
|
|
name="reason",
|
|
field=models.TextField(blank=True, null=True),
|
|
),
|
|
migrations.AddField(
|
|
model_name="motion",
|
|
name="modified_final_version",
|
|
field=models.TextField(blank=True, null=True),
|
|
),
|
|
migrations.AddField(
|
|
model_name="motion",
|
|
name="amendment_paragraphs",
|
|
field=jsonfield.fields.JSONField(
|
|
dump_kwargs={
|
|
"cls": jsonfield.encoder.JSONEncoder,
|
|
"separators": (",", ":"),
|
|
},
|
|
load_kwargs={},
|
|
null=True,
|
|
),
|
|
),
|
|
# Link change recommendations to motions directly
|
|
migrations.AddField(
|
|
model_name="motionchangerecommendation",
|
|
name="motion",
|
|
field=models.ForeignKey(
|
|
on_delete=django.db.models.deletion.CASCADE,
|
|
null=True, # This is reverted in the next migration
|
|
related_name="change_recommendations",
|
|
to="motions.Motion",
|
|
),
|
|
),
|
|
]
|